Ramp
Provide the Ramp functionality, including on-ramp and off-ramp, enabling buying and selling operations for ELF with fiat currencies.
Before using this component, please make sure you are logged-in.
Before using this component, we assume that you are already familiar with the basic configuration of Portkey.
Before using this component, we assume that you are already familiar with the basic configuration of Portkey.
Feature
Ramp's functions and UIs are as follows:
Buy
- Select the type and quantity of fiat currency for payment.
- Select the type of token to be received.
- Currently only supports ELF
- We will assist you in estimating the quantity to be received
- Exchange rates is renewed every 15 seconds with the estimated asset to be received also updated
- Provide limit amount prompts, allowing payment only in the specified range of fiat currency for token exchange.
Sell
- Select the type and quantity of tokens for payment.
- Currently only supports ELF.
- Select the type of fiat currency to be received
- We will assist you in estimating the quantity to be received
- Exchange rates is renewed every 15 seconds with the estimated asset to be received also updated
- Provide manage information synchronization prompt, transactions can only occur when manage information is present.
- Verify wallet security and transfer limits when users sell tokens
- Select the type and quantity of tokens for payment.
buying
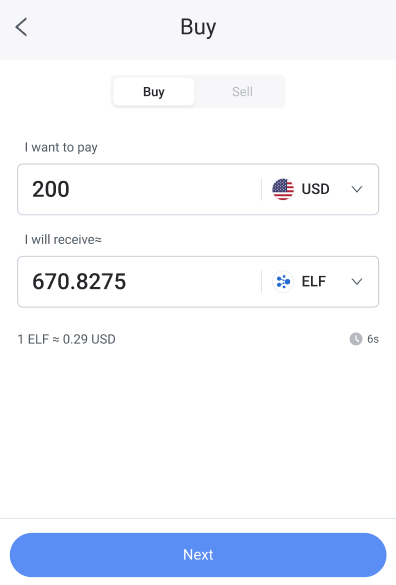
fiat currency selecting
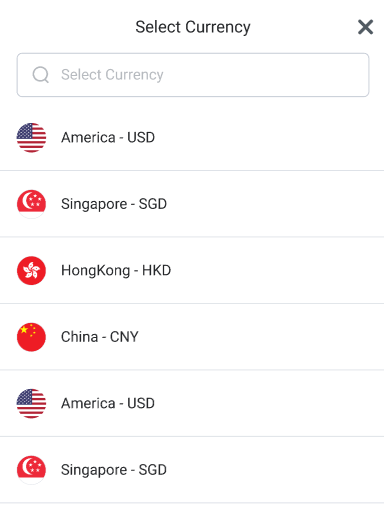
token selecting
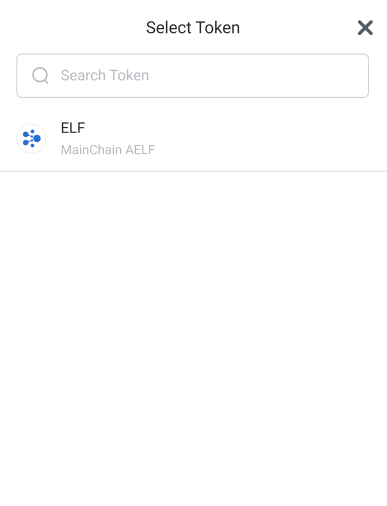
limit amount
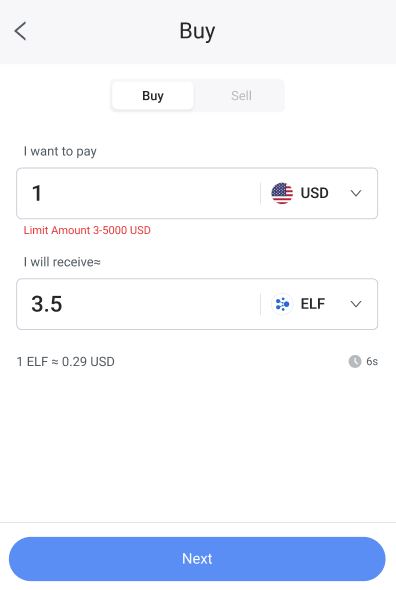
selling
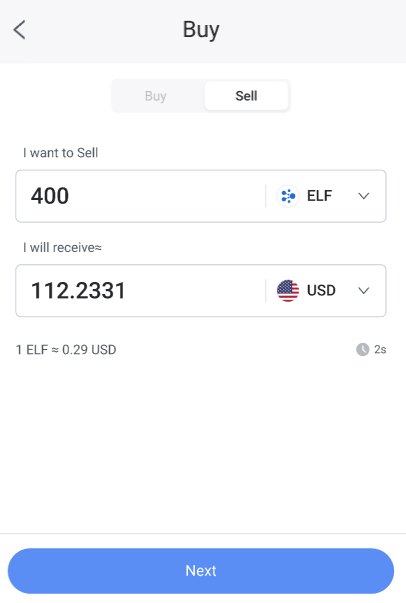
manage information synchronization
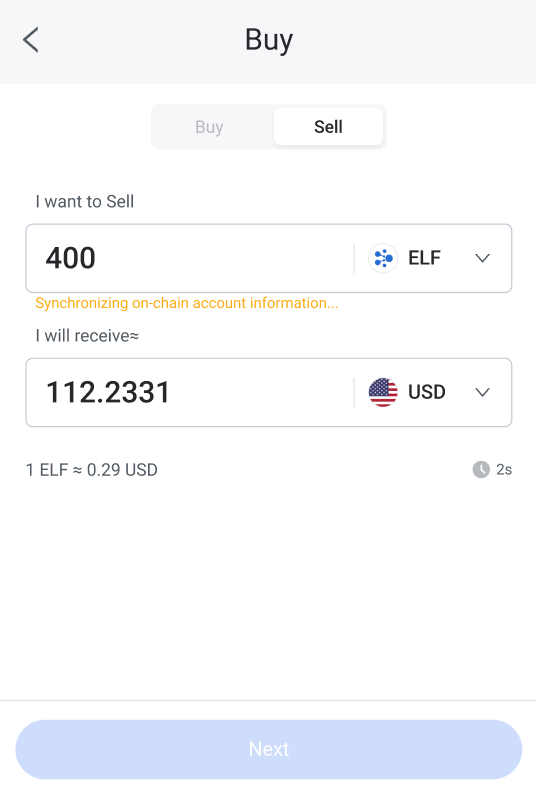
wallet security notice
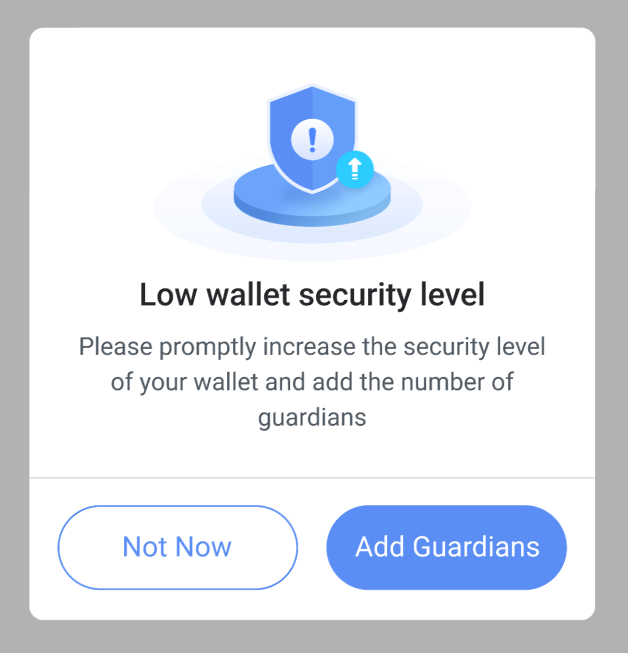
transfer limit notice
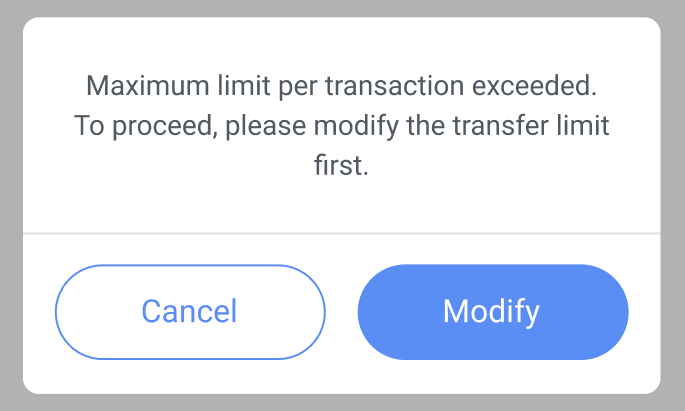
Usage
import { Ramp } from "@portkey/did-ui-react";
const App: React.FC = () => (
<Ramp
tokenInfo={{
decimals: 8,
chainId: "AELF",
symbol: "ELF",
tokenContractAddress: "JRmBduh4nXWi1aXgdUsj5gJrzeZb2LxmrAbf7W99faZSvoAaE",
}}
portkeyWebSocketUrl={"http://192.168.66.240:5577/ca"}
isMainnet={true}
onBack={function (): void {
// do something to show the last page
}}
onShowPreview={function ({ initState, chainId }): void {
// do something to show the preview transaction details page
}}
/>
);
export default App;
API
Property | Description | Type | Required | Default | Version |
---|---|---|---|---|---|
className | Class name of the top level container | string | false | — | |
initState | Data that controls the initial page display | IRampInitState | false | — | |
isBuySectionShow | Control if on-ramp is enabled | boolean | false | true | |
isSellSectionShow | Control if off-ramp is enabled | boolean | false | true | |
isMainnet | Is it Mainnet | boolean | false | — | |
tokenInfo | Token information | ITokenInfo | false | — | |
portkeyWebSocketUrl | WebSocket service address, requiring IP format | string | false | — | |
isErrorTip | Is error tip set as default | boolean | false | true | V2.0.0 |
onBack | Control the event to go back to the previous route | () => void | true | — | |
onShowPreview | Control the event to navigate to the preview | ({ initState, chainId }: { initState: IRampPreviewInitState; chainId: ChainId }) => void; | true | — |
Detailed explanation
IRampInitState
Page initialization data, you can define the default fiat currency and token information from here.
Interface | Property | Description | Type | Required |
---|---|---|---|---|
IRampInitState | ||||
crypto | Crypto name, eg: ELF | string | true | |
network | Crypto network eg: AELF | string | true | |
fiat | Fait code, eg: USD | string | true | |
country | Country code, eg: US | string | true | |
amount | Account number | string | false | |
side | Business type | strRampTypeEnum: "BUY" | "SELL" | true |
ITokenInfo
Current chain information for the operation, defaulting to aelf
Interface | Property | Description | Type | Required |
---|---|---|---|---|
ITokenInfo | ||||
decimals | Decimals, eg: 8 | number | string | true | |
chainId | Chain ID code, eg: 'AELF' | ChainId | true | |
symbol | Symbol code, eg: 'ELF' | string | true | |
tokenContractAddress | Token contract address | string | true |
IRampPreviewInitState
Parameters required to navigate to the Ramp preview page. Refer to RampPreview for details.
ChainId
Enumeration range: 'AELF' | 'tDVV' | 'tDVW';