Guardian
Usage scenario: guardian management
Detailed functions
- View guardian list
- Set or remove login guardian
- Add, edit, or remove guardian
UI
Display the guardian list of the current account
Example: a list that contains four types of guardians, i.e. Google account, Telegram, Apple ID, and email.
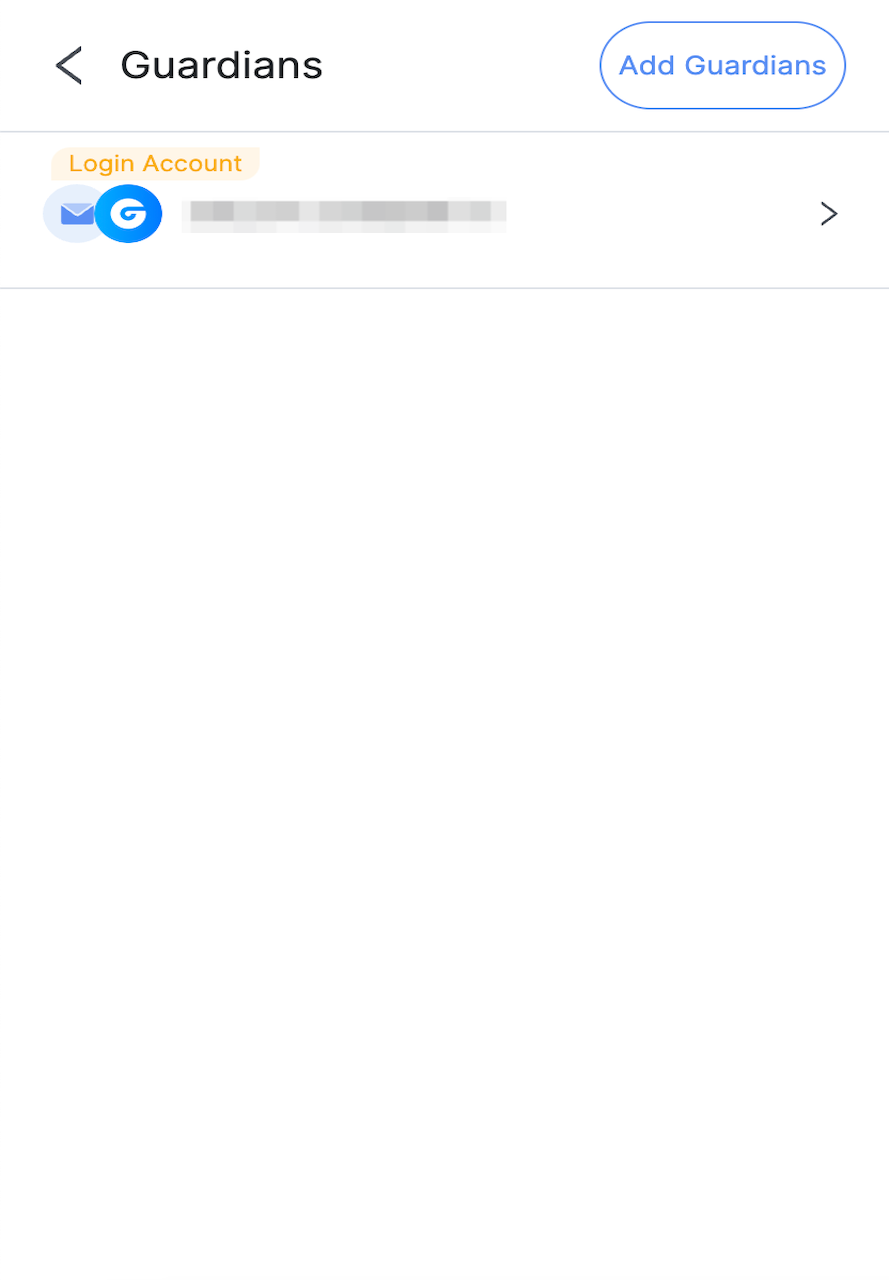
Add guardians
- Select guarfian type: Google account, Telegram, Apple ID, and email
- Select verifier
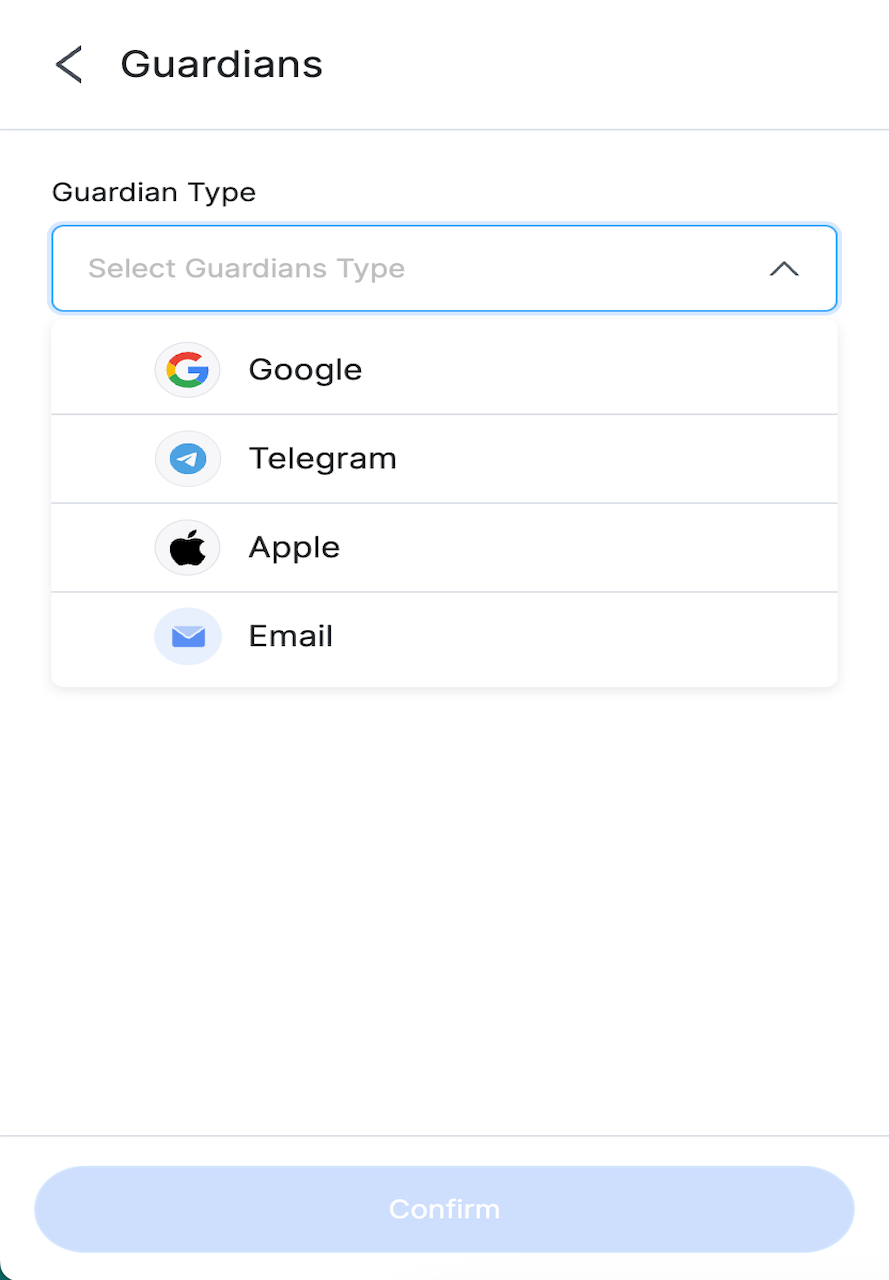
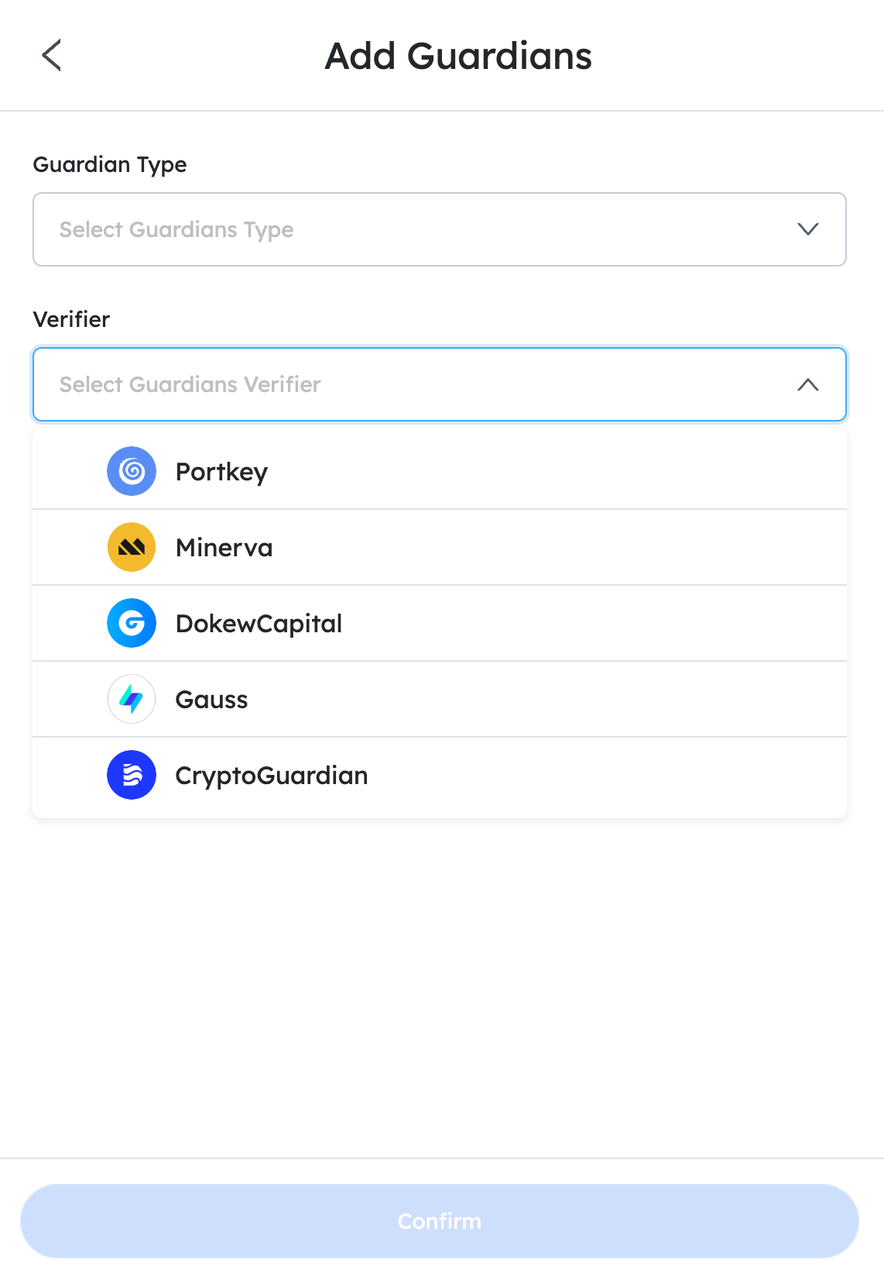
Guardian details
Set the current guardian as a login guardian or remove it
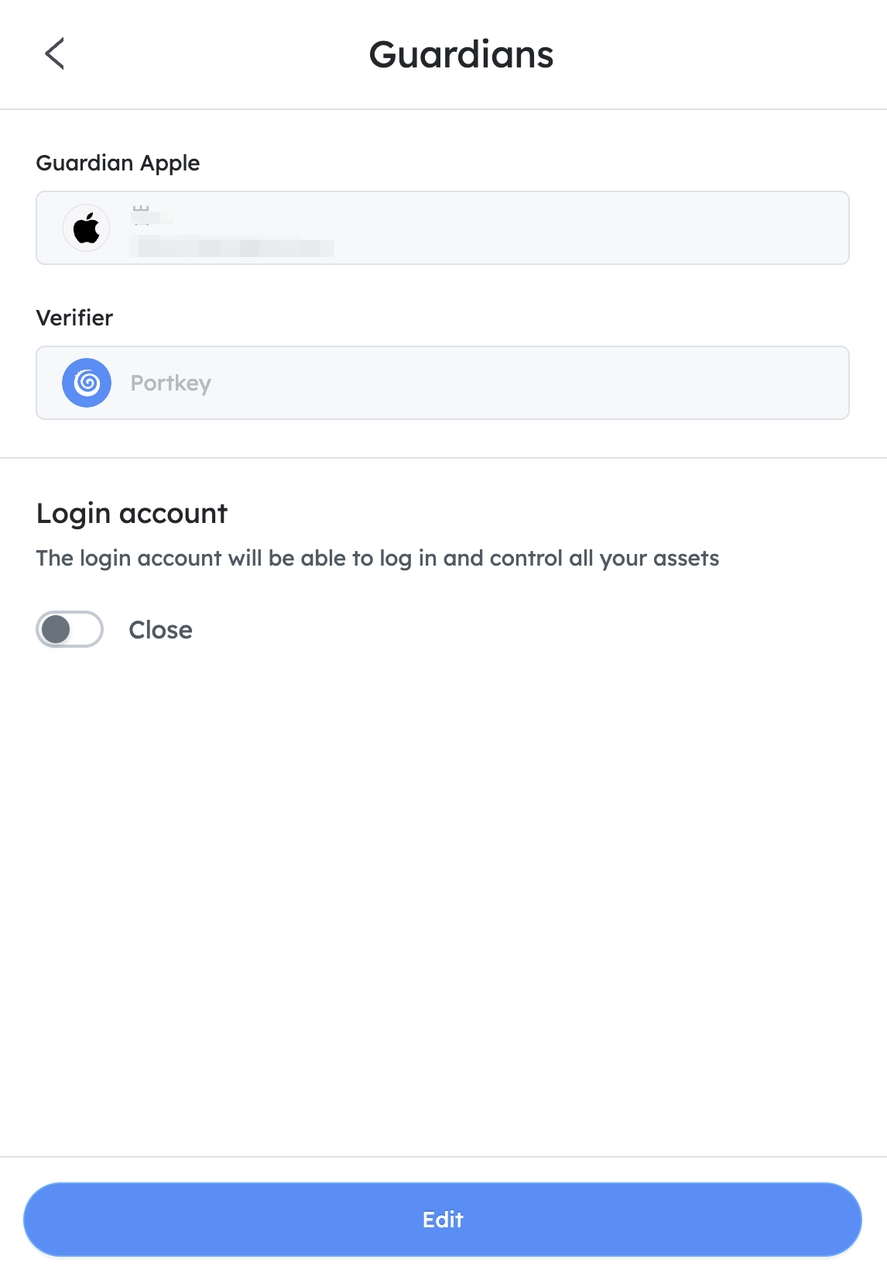
Edit Guardians
- Can only change the verifier of the guardian
- Remove the guardian
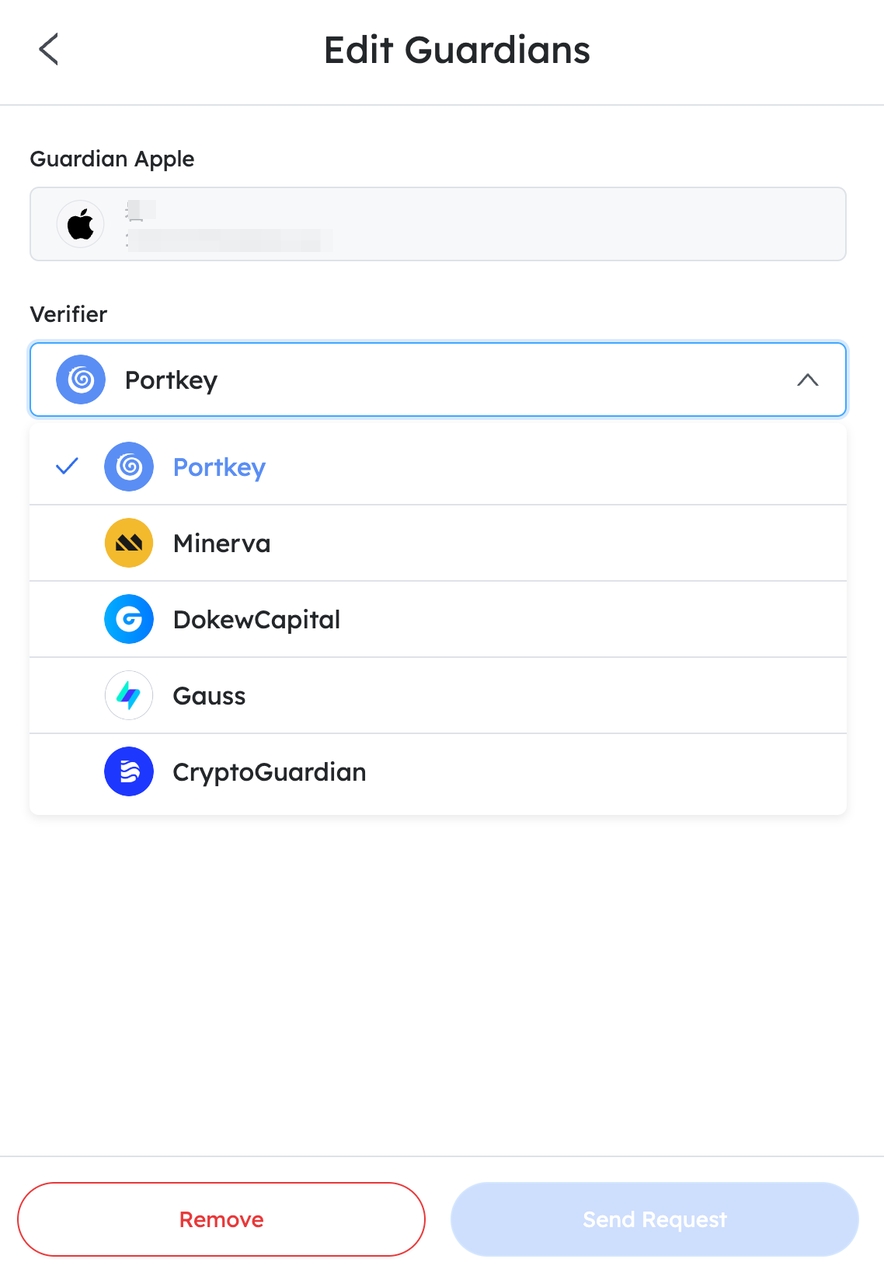
Guardian
Manage the current account's guardians, integrating GuardianPageList, GuardianAdd, GuardianView, and GuardianEdit
Usage
import { Guardian } from "@portkey/did-ui-react";
const App = () => (
<Guardian
caHash={"xxxxxxx"}
originChainId={"AELF"}
accelerateChainId={"tDVV"}
onBack={() => {
// back callback
}}
networkType="MAINNET"
/>
);
export default App;
API
Property | Description | Type | Default | Version |
---|---|---|---|---|
caHash | caHash of the Portkey account | string | - | V1.5.1 |
className | Customised class name | string | - | V1.5.1 |
originChainId | ID of the chain where user account is registered | ChainId | - | V1.5.1 |
accelerateChainId | ID of the chain where synchronisation of guardian is accelerated, done by initiating a transaction to quickly add the guardian | ChainId | - | V1.5.1 |
chainType | ChainType | aelf | V1.5.1 | |
isErrorTip | Is error tip set as default | boolean | true | V1.5.1 |
onError | Callback of error | (error: {errorFields:string, error: any}) => void | - | V1.5.1 |
onBack | Callback of back | () => void | - | V1.5.1 |
networkType | Network type | MAINNET | TESTNET | - | V2.0.0 |
Guardian Page List
Dsiplay the guardian list of the current account
Usage
import { GuardianPageList } from "@portkey/did-ui-react";
const App = () => (
<GuardianPageList
header={<div>Guardian List Header</div>}
guardianList={[
{
isLoginGuardian: true,
verifier: {
endPoints: ["your endPoints"],
verifierAddresses: ["your verifierAddresses"],
id: "your verifier id",
name: "your verifier name",
imageUrl: "your verifier imageUrl",
},
identifier: "identifier",
guardianType: "Phone",
key: "",
},
]}
onViewGuardian={(item: UserGuardianStatus) => {
// view guardian item
}}
/>
);
export default App;
API
Property | Description | Type | Default | Version |
---|---|---|---|---|
header | Header UI component | ReactNode | - | V1.5.1 |
className | Customised class name | string | - | V1.5.1 |
guardianList | Guardian list of the current account | UserGuardianStatus[] | - | V1.5.1 |
tipContainer | Customized content | ReactNode | - | V2.0.0 |
onViewGuardian | Callback when guardian item is clicked | (item: UserGuardianStatus) => void | - | V1.5.1 |
Guardian Add
Add guardian
Usage
import { GuardianAdd } from '@portkey/did-ui-react';
const verifierList = [{
id: "594ebf...94b76b"
imageUrl: "https://xxx/Portkey.png"
name: "Portkey"
}];
const guardianList = [
{
isLoginGuardian: true,
verifier: {
endPoints: ['your endPoints'],
verifierAddresses: ['your verifierAddresses'],
id: 'your verifier id',
name: 'your verifier name',
imageUrl: 'your verifier imageUrl',
},
identifier: 'identifier',
guardianType: 'Phone',
key: '',
},
];
const App = () => (
<GuardianAdd
header={<div>Guardian Add Header</div>}
originChainId="AELF"
verifierList={verifierList}
guardianList={guardianList}
handleAddGuardian={function() {
// to add guardian
}}
/>
);
export default App;
API
Property | Description | Type | Default | Version |
---|---|---|---|---|
header | Header UI component | ReactNode | - | V1.5.1 |
className | Customised class name | string | - | V1.5.1 |
caHash | caHash of the Portkey account | string | - | V2.0.0 |
originChainId | ID of the chain where user account is registered | ChainId | - | V1.5.1 |
chainType | ChainType | - | V2.0.0 | |
phoneCountry | Country calling code | IPhoneCountry | - | V1.5.1 |
guardianList | Guardian list of the current account | UserGuardianStatus[] | - | V1.5.1 |
verifierList | List of verifiers supported | VerifierItem[] | - | V1.5.1 |
networkType | Network type | MAINNET | TESTNET | - | V2.0.0 |
sandboxId | string | - | V2.0.0 | |
isErrorTip | Is error tip set as default | boolean | true | V1.5.1 |
onError | Callback of error | (error: {errorFields:string, error: any}) => void | - | V1.5.1 |
handleAddGuardian | Execute contract method that adds guardian | (currentGuardian: UserGuardianStatus, approvalInfo: GuardiansApproved[]) => Promise\<any> | - | V1.5.1 |
Guardian View
View guardian item
Usage
import { GuardianView } from "@portkey/did-ui-react";
const currentGuardian = {
isLoginGuardian: true,
verifier: {
endPoints: ["your endPoints"],
verifierAddresses: ["your verifierAddresses"],
id: "your verifier id",
name: "your verifier name",
imageUrl: "your verifier imageUrl",
},
identifier: "identifier",
guardianType: "Phone",
key: "",
};
const guardianList = [
{
isLoginGuardian: true,
verifier: {
endPoints: ["your endPoints"],
verifierAddresses: ["your verifierAddresses"],
id: "your verifier id",
name: "your verifier name",
imageUrl: "your verifier imageUrl",
},
identifier: "identifier",
guardianType: "Phone",
key: "",
},
];
const App = () => (
<GuardianView
networkType="MAINNET"
header={<div>Guardian View Header</div>}
currentGuardian={currentGuardian}
handleSetLoginGuardian={async (
currentGuardian: UserGuardianStatus,
approvalInfo: GuardiansApproved[]
) => {
// operate set login guardian
}}
guardianList={guardianList}
/>
);
export default App;
API
Property | Description | Type | Default | Version |
---|---|---|---|---|
header | Header UI component | ReactNode | - | V1.5.1 |
className | Customised class name | string | - | V1.5.1 |
originChainId | ID of the chain where user account is registered | ChainId | - | V1.5.1 |
currentGuardian | Current guardian item | UserGuardianStatus | - | V1.5.1 |
guardianList | Guardian list of the current account | UserGuardianStatus[] | - | V1.5.1 |
networkType | Network type | MAINNET | TESTNET | - | V2.0.0 |
isErrorTip | Is error tip set as default | boolean | true | V1.5.1 |
onError | Callback of error | (error: {errorFields:string, error: any}) => void | - | V1.5.1 |
onEditGuardian | Execute contract method that edits guardian | () => void | - | V1.5.1 |
handleSetLoginGuardian | Execute contract method that sets or removes guardian | (currentGuardian: UserGuardianStatus, approvalInfo: GuardiansApproved[]) => Promise\<any> | - | V2.0.0 |
Guardian Edit
Edit guardian item
Usage
import { GuardianEdit } from '@portkey/did-ui-react';
const verifierList = [{
id: "594ebf...94b76b"
imageUrl: "https://xxx/Portkey.png"
name: "Portkey"
}];
const currentGuardian = {
isLoginGuardian: true,
verifier: {
endPoints: ['your endPoints'],
verifierAddresses: ['your verifierAddresses'],
id: 'your verifier id',
name: 'your verifier name',
imageUrl: 'your verifier imageUrl',
},
identifier: 'identifier',
guardianType: 'Phone',
key: '',
};
const guardianList = [
{
isLoginGuardian: true,
verifier: {
endPoints: ['your endPoints'],
verifierAddresses: ['your verifierAddresses'],
id: 'your verifier id',
name: 'your verifier name',
imageUrl: 'your verifier imageUrl',
},
identifier: 'identifier',
guardianType: 'Phone',
key: '',
},
];
const preGuardian = {
isLoginGuardian: true,
verifier: {
endPoints: ['your endPoints'],
verifierAddresses: ['your verifierAddresses'],
id: 'your verifier id',
name: 'your verifier name',
imageUrl: 'your verifier imageUrl',
},
identifier: 'identifier',
guardianType: 'Phone',
key: '',
};
const App = () => (
<GuardianEdit
caHash="cahash"
networkType="MAINNET"
header={<div>Guardian Edit Header</div>}
verifierList={verifierList}
currentGuardian={currentGuardian}
guardianList={guardianList}
preGuardian={preGuardian}
handleEditGuardian={async (currentGuardian: UserGuardianStatus, approvalInfo: GuardiansApproved[]) => {
// update current guardian
}}
handleRemoveGuardian={async (approvalInfo: GuardiansApproved[]) => {
// remove current guardian
}}
handleSetLoginGuardian={async (currentGuardian: UserGuardianStatus, approvalInfo: GuardiansApproved[]) => {
// set or unset login guardian
}}
/>
);
export default App;
API
Property | Description | Type | Default | Version |
---|---|---|---|---|
header | Header UI component | ReactNode | - | V1.5.1 |
className | Customised class name | string | - | V1.5.1 |
originChainId | ID of the chain where user account is registered | ChainId | - | V1.5.1 |
caHash | caHash of the Portkey account | string | - | V2.0.0 |
chainType | ChainType | aelf | V2.0.0 | |
sandboxId | string | - | V2.0.0 | |
networkType | Network type | MAINNET | TESTNET | - | V2.0.0 |
currentGuardian | Guardian item after the edit | UserGuardianStatus | - | V1.5.1 |
preGuardian | Current guardian item | UserGuardianStatus | - | V1.5.1 |
guardianList | Guardian list of the current account | UserGuardianStatus[] | - | V1.5.1 |
verifierList | List of verifiers supported | VerifierItem[] | - | V1.5.1 |
isErrorTip | Is error tip set as default | boolean | true | V1.5.1 |
onError | Callback of error | (error: {errorFields:string, error: any}) => void | - | V1.5.1 |
handleEditGuardian | Execute contract method that edits guardian | (currentGuardian: UserGuardianStatus, approvalInfo: GuardiansApproved[]) => Promise\<any> | - | V1.5.1 |
handleRemoveGuardian | Execute contract method that removes guardian | (approvalInfo: GuardiansApproved[]) => Promise\<any> | - | V1.5.1 |
handleSetLoginGuardian | Execute contract method that sets or cancels guardian | (currentGuardian: UserGuardianStatus, approvalInfo: GuardiansApproved[]) => Promise\<any>; | - | V2.0.0 |